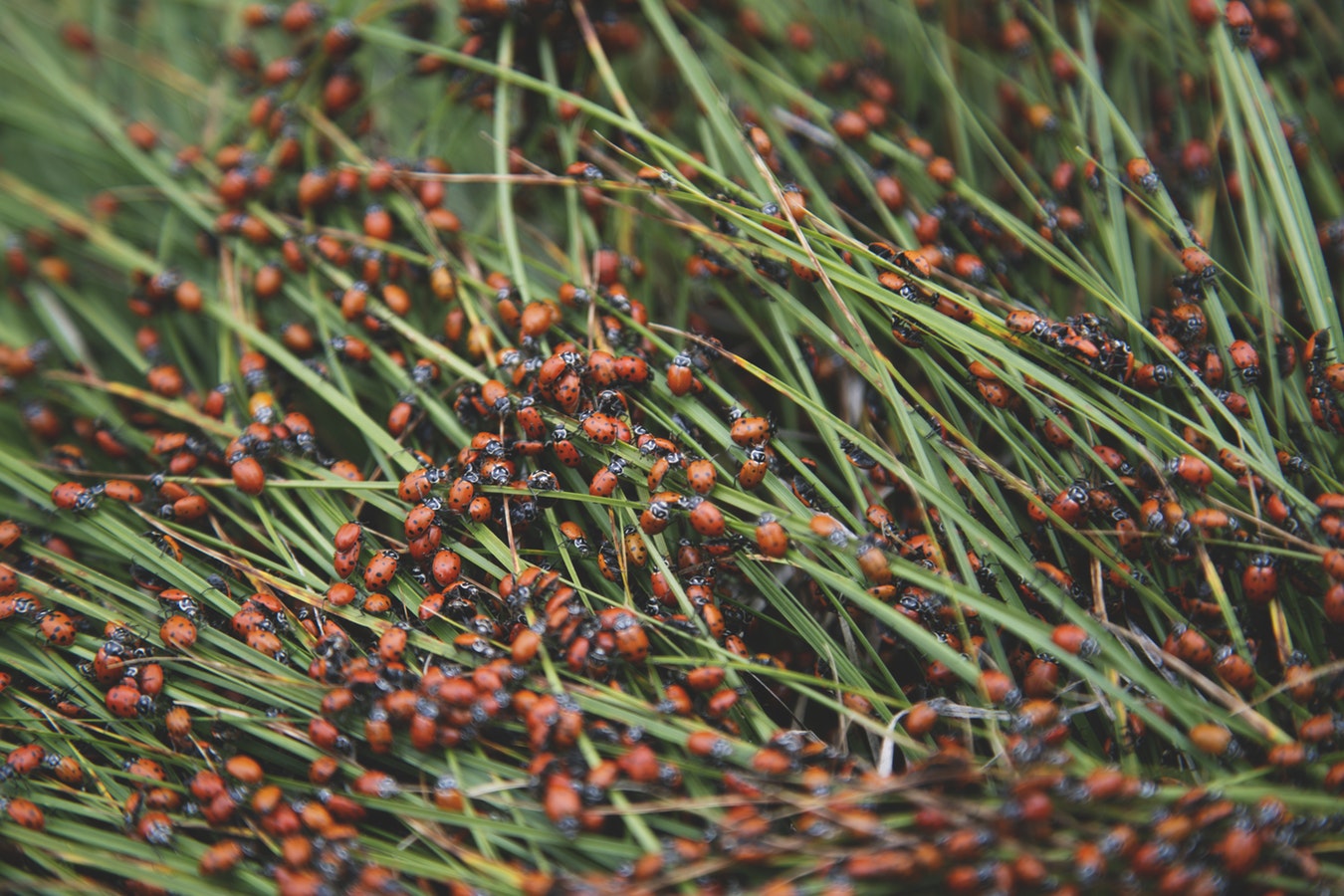
Error logging can be a challenge sometimes. Depending on what type server you are running, or if you are doing pure front-end javascript application without direct server access, your options for logging and analyzing application errors will vary greatly. What we are going to present to you today is a handy alternative to capturing client-side javascript errors that works for applications without server access and you can use if for free, since Google Analytics offers a very liberal free quota.
The other benefits of this approach are that it is very simple to implement and to use, and you can readily use the dashboard options provided by GA out-of-the box without needing to build fancy custom dashboards for analyzing errors. If you are very handy with setting up web hooks, there may be ways to setup web hooks for analytics events to receive notifications of critical issues in real-time, although we have never explored this angle.
The downside of this approach is that since some users disable all analytics tracking, you may not get 100 % complete view of all issues and their frequency. There are also some frequency limits of how many events GA will capture each second/minute but unless you have an incredibly popular website, this should not be a major consideration.
Prerequisites
You must have gtag.js
installed to use this code snippet. Follow the instructions provided here if you do not have it installed yet.
The Code
Insert this code snippet somewhere on your page one time. Depending on where you are injecting the snippet, you may omit the surrounding <script/>
tags.
1 | <script> |
The Code explained
1 | window.addEventListener('error', function (e) { ... }); |
This first line will attach an error listener to the browser window. It will listen to any errors raised by your application during its runtime. If you have a single page app, make sure to only register this listener one time so as to not fire duplicate error entries to your log. If you are using server-side rendered web pages, make sure to include this in the template you return for each page.
1 | var errorText = [ |
This variable is used to store details about the error that occurred. It captures the specific error message, line number, column number, the name of the script file and stack trace (if exists). Lastly all this information is joined into a string, where each distinct line is separated by a line break.
1 | window.gtag('event', 'log', { |
Finally we need to send the captured information to Google Analytics. If you are not using gtag (but analytics.js) the syntax for posting an event to GA is a little bit different and you will have to check the documentation for the exact syntax.
After adding this code snippet, we recommend you purposely take action that throws a javascript error to ensure the code is working correctly for you. In your analytics console, you can observer events in real time by navigating to Real-Time > Events > Events (Last 30 min)
. If you are using a filters to exclude local traffic, make sure to debug in an environment where the events are not filtered.
Happy Coding!